Heightmaps – Introduction
A heightmap is an image representing variations in height relative to a surface.
Generally, a heightmap is composed of shades of gray : White represents the highest heights, and black, the lowest.
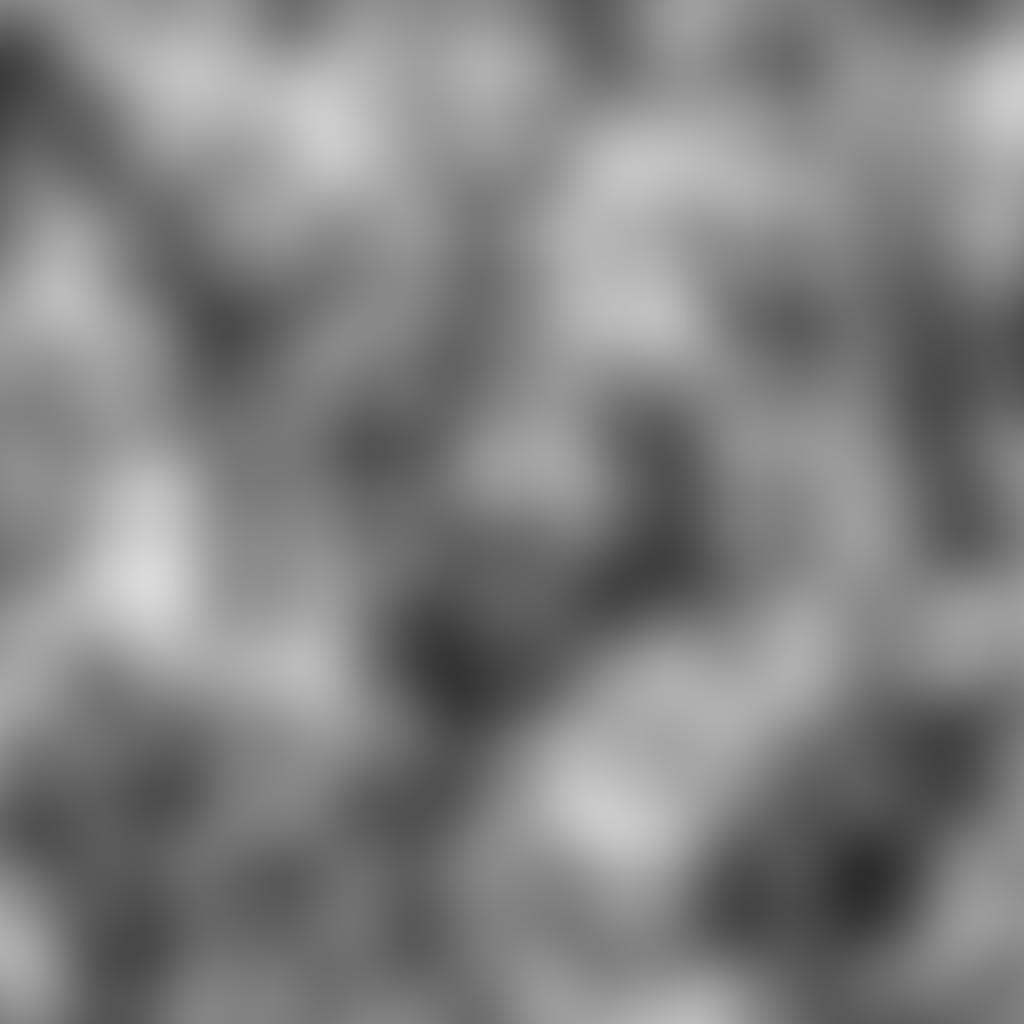
Using a heightmap in Three.js
We can use a heightmap to create a complex terrain in our Three.js scene !
To do this, let’s start by creating a Mesh
based on a PlaneGeometry
instance :
const planeGeometry = new THREE.PlaneGeometry(100, 100, 50, 50); const planeMaterial = new THREE.MeshPhongMaterial( { color : 0xFFFFFF, side: THREE.DoubleSide }); const mesh = new THREE.Mesh(planeGeometry, planeMaterial); mesh.rotation.x = Math.PI /2; scene.add(mesh);
We will use the heightmap to deform our 3D object, so it is important that the structure of our PlaneGeometry
is complex enough.
As a reminder, parameters 3 and 4 of the PlaneGeometry
constructor are used to define the complexity of the structure : new THREE.PlaneGeometry(100, 100, 50, 50);
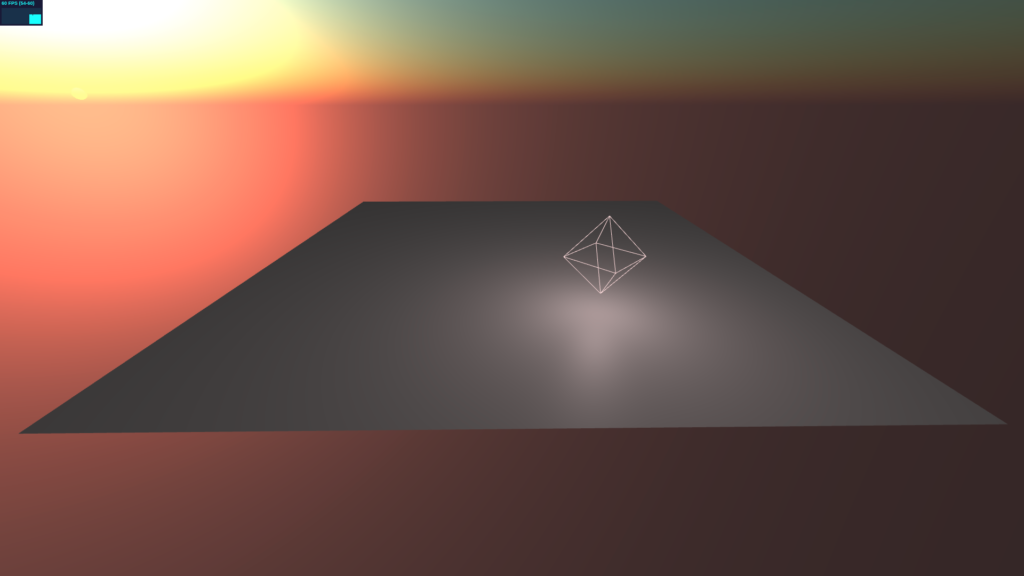
Then, let’s load the heightmap with TextureLoader
:
var heightMap = new THREE.TextureLoader().load("heightmap.png");
When this is done, we are going to use the displacementMap
and displacementScale
properties of our Material
:
var heightMap = new THREE.TextureLoader().load("heightmap.png"); const planeGeometry = new THREE.PlaneGeometry(100, 100, 100, 100); const planeMaterial = new THREE.MeshPhongMaterial( { color : 0xFFFFFF, side: THREE.DoubleSide, displacementMap : heightMap, displacementScale : 20 }); const mesh = new THREE.Mesh(planeGeometry, planeMaterial); mesh.rotation.x = Math.PI /2; scene.add(mesh);
The displacementMap
property allows to link the Material
and the heightmap. Regarding displacementScale
, it allows you to define the intensity of application of the heightmap ( to be adapted according to your projects and needs).
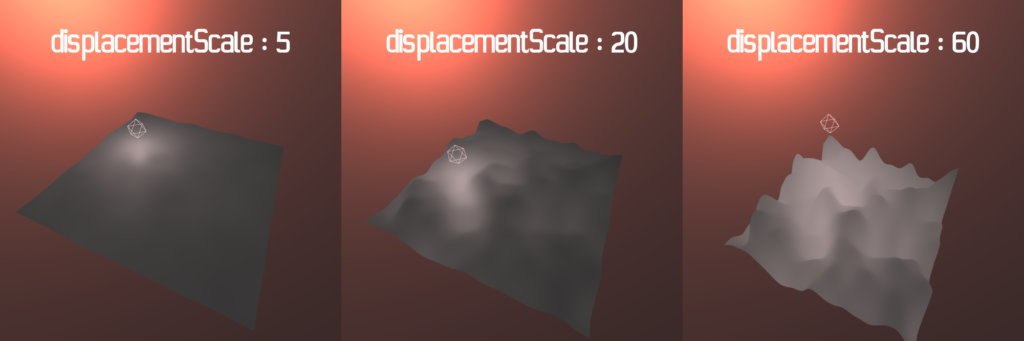
displacementScale
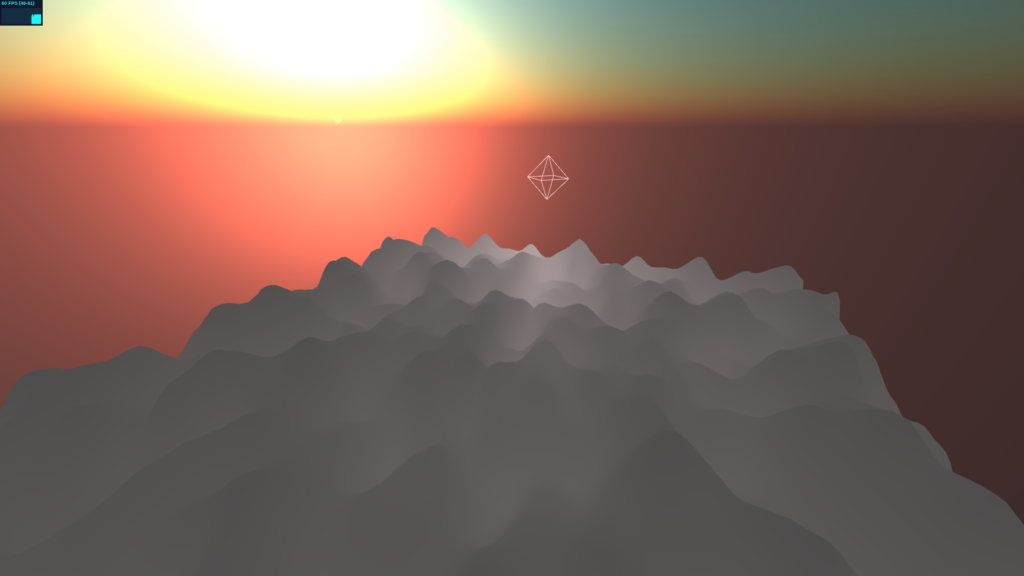
Note that it is possible to use heightmaps with any type of Geometry
:
var heightMap = new THREE.TextureLoader().load("heightmap_seamless.png"); const sphereGeo = new THREE.SphereGeometry(15, 512, 512); const planeMaterial = new THREE.MeshPhongMaterial( { color : 0xFFFFFF, side: THREE.DoubleSide, displacementMap : heightMap, displacementScale : 20 }); const mesh = new THREE.Mesh(sphereGeo, planeMaterial); mesh.rotation.x = Math.PI /2; scene.add(mesh);
( Get a seamless heightmap texture ! )
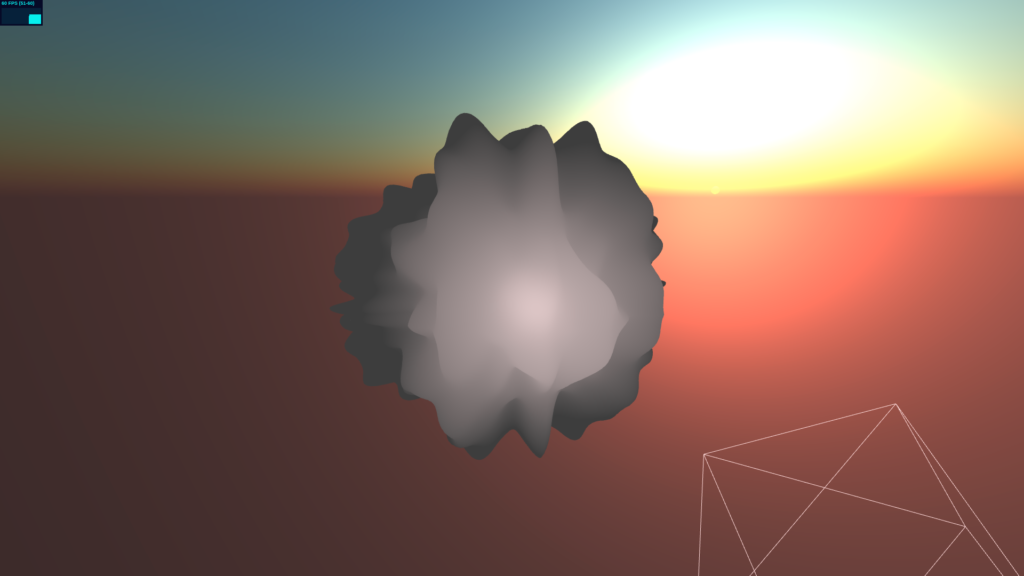
SphereGeometry
Now you can use a heightmap to easily create a Three.js terrain !
[…] thomassifferlen Available in English […]