Executing a 3D rendering at 60 Frames per Second (FPS) in our Three.js application is a guarantee for a fluid and enjoyable experience. Nevertheless, it’s an objective that’s sometimes difficult to achieve !
During the writing of my examples and tutorials, I often face a performance problem when I test my creations on older or lower-end devices.
I compiled in this article my best tips and tricks for optimizing your Three.js application and for reaching 60 FPS; Happy reading!
Before You Start
Measuring performances
Before anything else, we must be capable of measuring the performances of our application. I suggest you use the module stats
available on GitHub.
This little library allows for the addition of a performance monitor on our page :
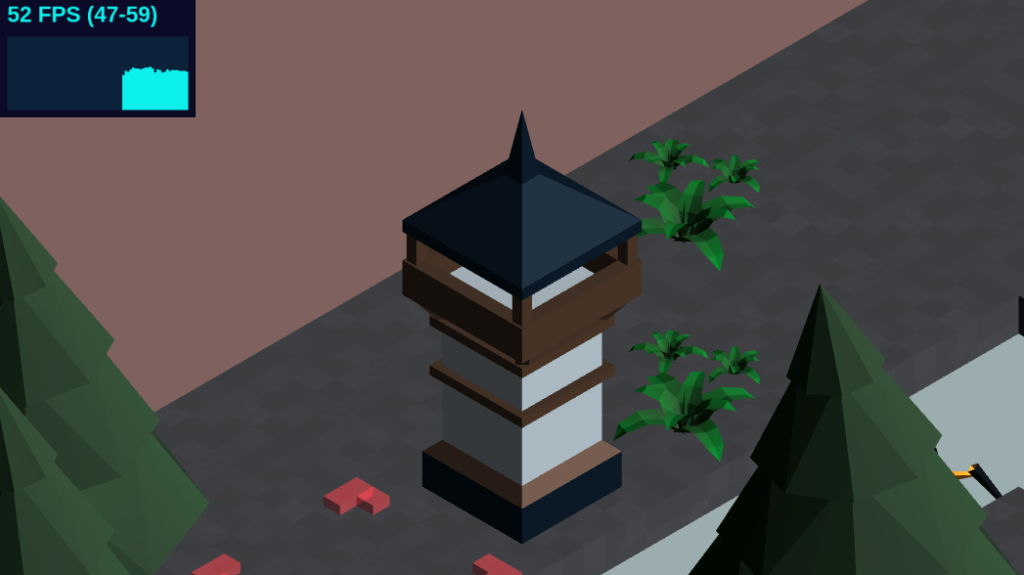
stats
– Here, our application Three.js runs at 52 FPSThe choice of web browser
As you know, our Three.js applications are written in JavaScript – an interpreted language. Our code will therefore be directly executed by the JavaScript engine of the browser.
There exists many web browsers on the market; the speed of execution of our code is therefore strongly linked to the web browser that executes it !
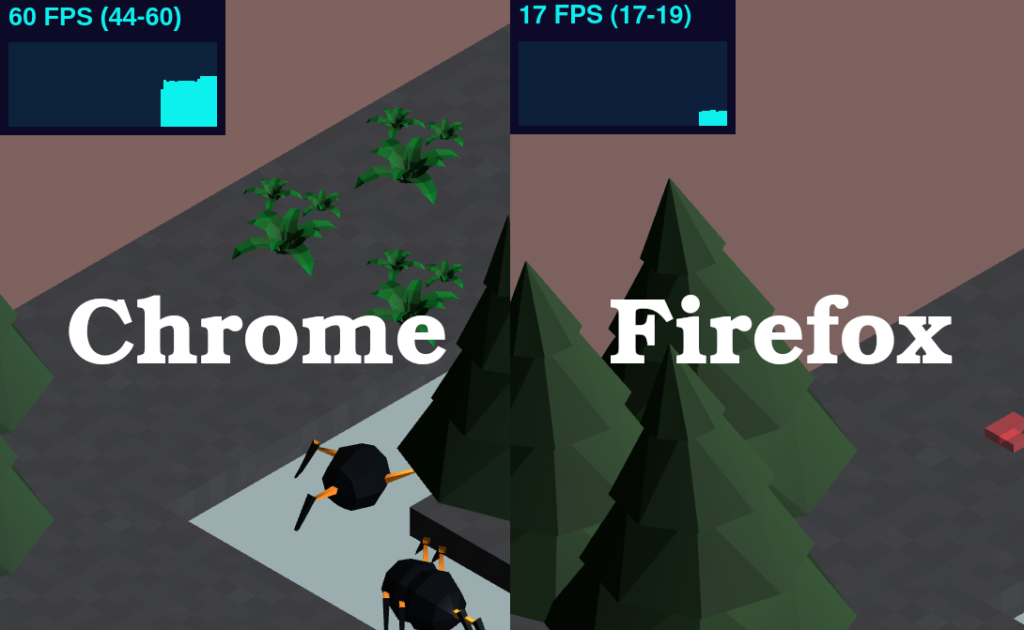
Google Chrome is reputed for performing very well with WebGL and Three.js. This is the reason why it’s important to work with several navigators, thereby assuring us that the level of global optimization on the market is satisfactory.
A browser with the most recent updates also generally has the best performance.
Reducing the number of polygons in the scene
The first method of optimization concerns the number of polygons in the scene.
During each execution of the 3D rendering, the processor is charged with displaying the objects of the scene. The more polygons (faces of the 3D object’s structure) there are to process, the more significant the load of work shall be.
It is possible to simply display the number of polygons currently processed by the rendering engine with this line of code :
console.log("Number of Triangles :", renderer.info.render.triangles);
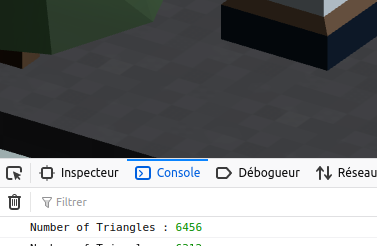
Thus, a good means of augmenting the performances of our application is to reduce the complexity of the scene. For this, we have two approaches :
- Removing 3D objects from the scene
- Simplifying the 3D objects by reducing their number of polygons
In the first case, we must simply triage and remove certain 3D objects from the scene.
In the second case, the work is more minutious. With the help of our 3D modeling software, we will simplify our models and remove as many faces as possible. It is for example possible to remove all the faces hidden and not visible by the camera.
Certain softwares such as Blender even offer functionalities for automatic simplification of 3D structures !
Deactivating anti-aliasing
Deactivating anti-aliasing in the rendering motor is an easy way to seriously boost the performance of our application in exchange of some visible pixels.
Effectively, anti-aliasing is used to smooth out the contours of a 3D object and give a neater finish in the final rendering – very efficient, but increase the processor’s workload !
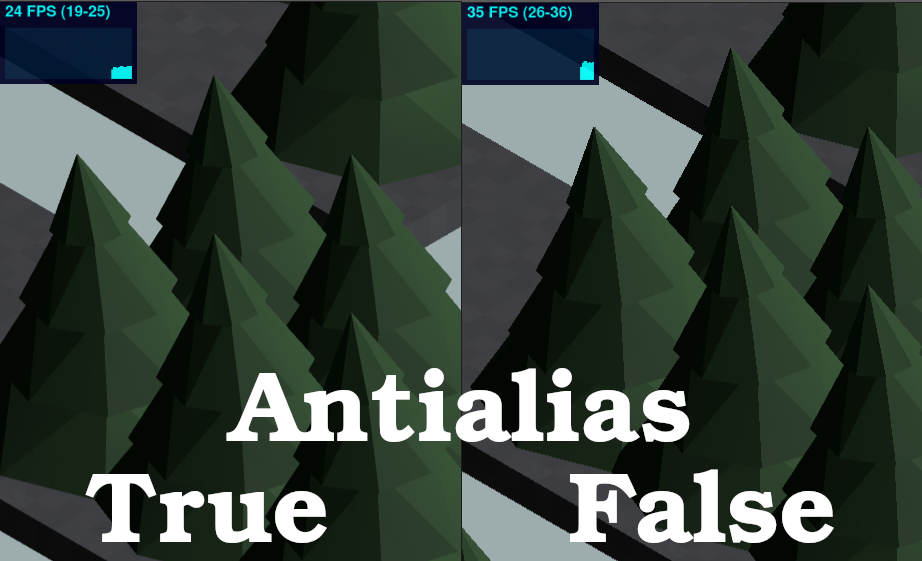
To activate or deactivate anti-aliasing, we use the property antialias of our 3D rendering engine :
//Enabled renderer = new THREE.WebGLRenderer( { antialias : true } ); //--------------------- //Disabled renderer = new THREE.WebGLRenderer( { antialias : false } );
Deactivating anti-aliasing is a simple and effective way to optimize the performance of our Three.js application!
Reducing the resolution of the 3D Rendering
Reducing the resolution ( number of calculated pixels ) during the 3D rendering is another way to easily optimize our Three.js application.
This method is extremely effective, but the loss in graphic quality is significant. Thus, I suggest you use this method as a last resort !
To reduce the resolution, we will use the method setPixelRation
of our 3D rendering engine.
//Base value renderer.setPixelRatio( window.devicePixelRatio );
By modifying the pixel ratio
, it is possible to diminish the number of calculated pixels, thereby modifying the final resolution !
Therefore, let’s modify the resolution of our 3D rendering with a factor of 0.5
:
//Lower résolution renderer.setPixelRatio( window.devicePixelRatio * 0.5 );
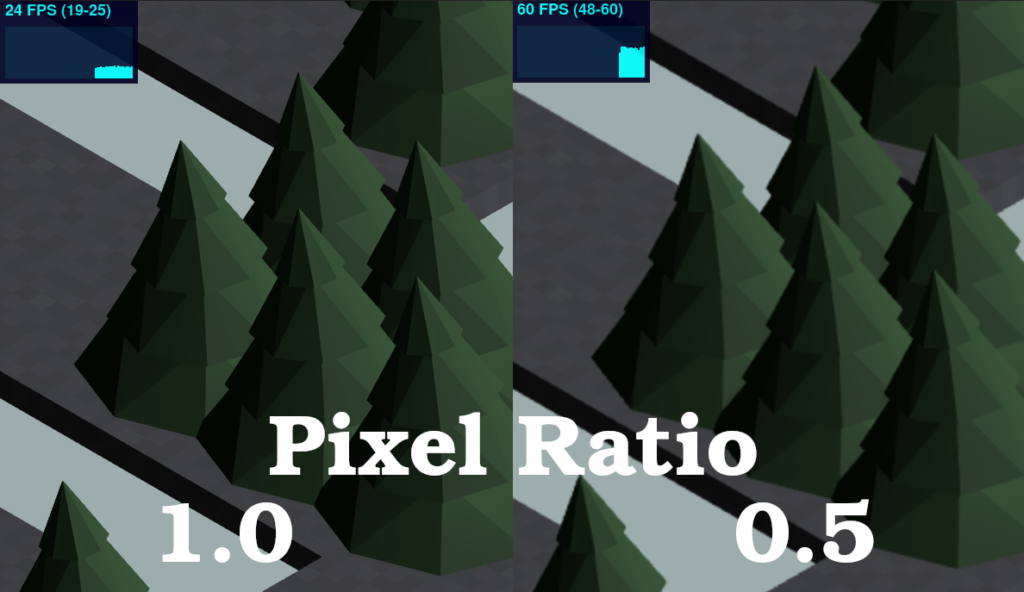
As you may have noticed, the gains in FPS are impressive (from 24 FPS to 60 FPS), but the graphic quality has greatly decreased.
Note that it is possible to adjust this value; a pixel ratio
from 0.8
will be much less visible graphics-wise than 0.5
, but less efficient for gaining FPS !
Feel free to download the complete Three.js University guide to progress even faster !
Still haven’t reached your 60 FPS goal ? Want to optimize your application even more ?
In the Bonus chapter of the downloadable guide, we’ll discover even more Three.js optimization techniques and tips !
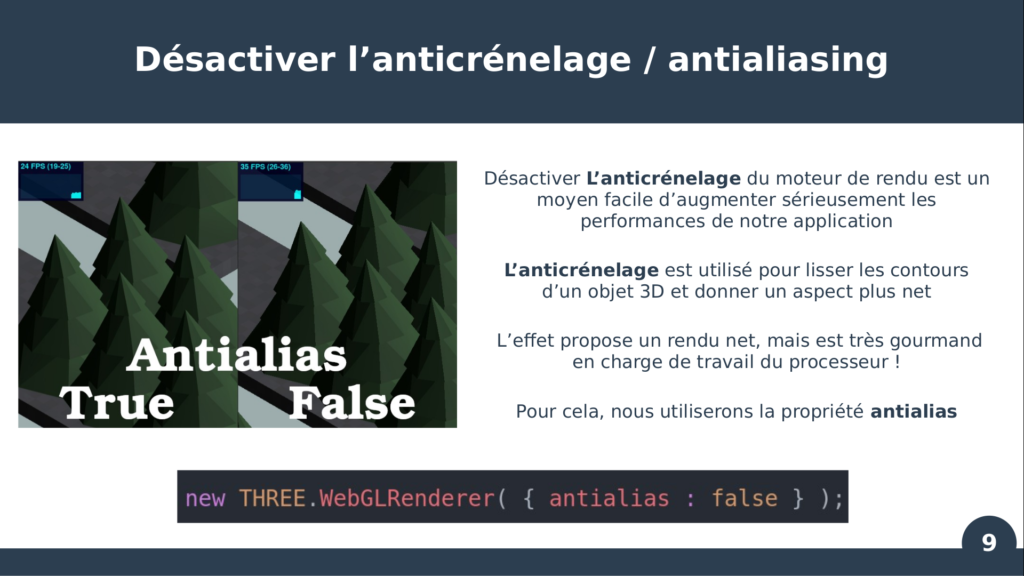
You can download the complete guide here :
On Mac Chrome is not at all a speedy webGL browser. The latest Firefox is the fastest followed by Safari and Chrome basically sucks.
Thanks for your comment ! 🙂
It looks like it’s really hard to predict performance with all these parameters haha
[…] thomassifferlen 1 commentaire Available in English […]