To create shadows in Three.js, we need a few things :
- A rendering engine capable of creating shadows
- An appropriate lighting
- A 3D object capable of creating a shadow
- A 3D object capable of receiving a shadow
The shadowMap.enabled property of WebGLRenderer
Our 3D renderer shall be able to handle shadows ! For this, it is necessary to set the shadowMap.enabled
property of our WebGLRenderer
to true
.
// ---------------- RENDERER ---------------- renderer = new THREE.WebGLRenderer( { antialias : true } ); renderer.setPixelRatio( window.devicePixelRatio ); renderer.setSize( window.innerWidth, window.innerHeight ); renderer.shadowMap.enabled = true; // IMPORTANT FOR SHADOWS !!! document.body.appendChild( renderer.domElement );
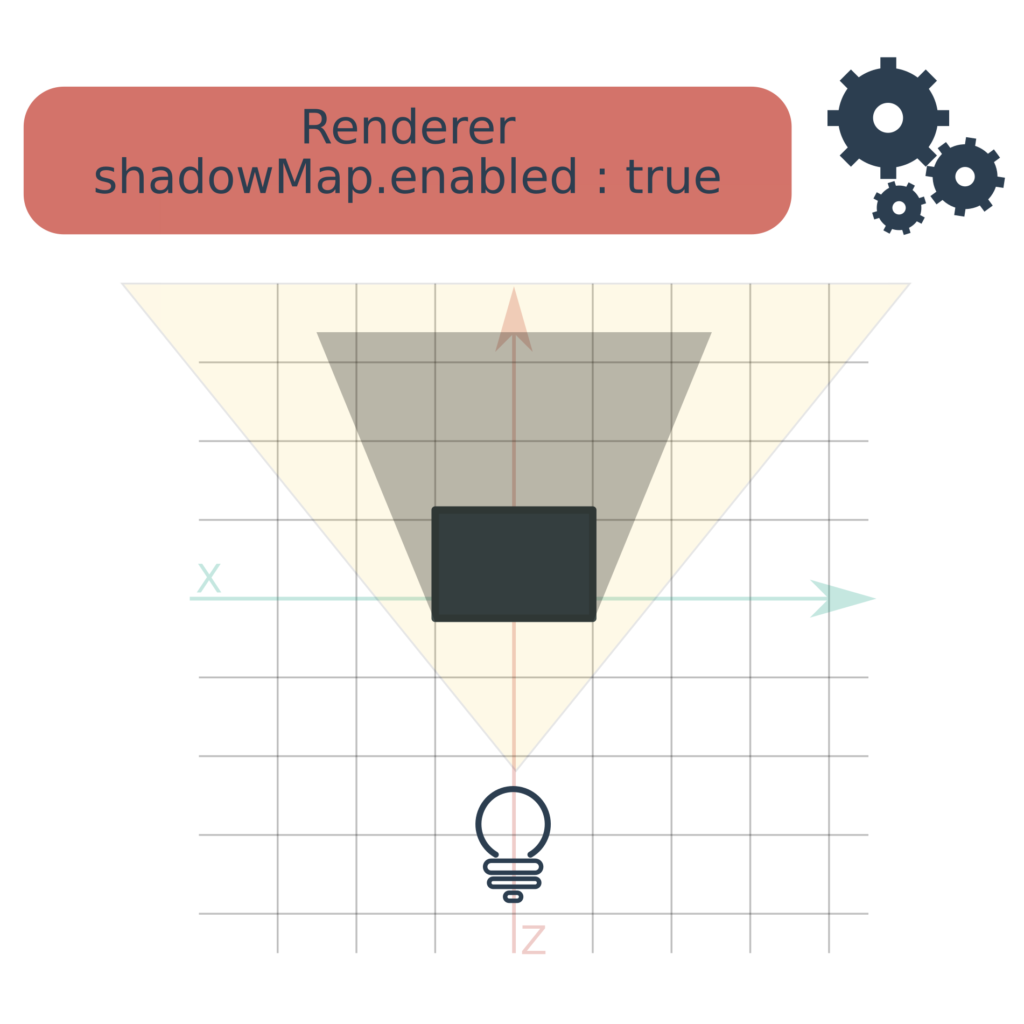
Choose and configure lighting
To create shadows, we must use appropriate lighting.
Indeed, not all lights are able to create shadows ! In this example we will use the PointLight
class.
Note that the
DirectionalLight
class of our Hello World can also create shadows !
//POINTLIGHT , FOR SHADOWS const color = 0xFFFFFF; const intensity = 0.5; light = new THREE.PointLight(color, intensity); light.castShadow = true; // IMPORTANT FOR SHADOWS !!! light.position.set(0, 10, 0); scene.add(light);
We set the castShadow
property of our lighting to true
, to allow it to create shadows.
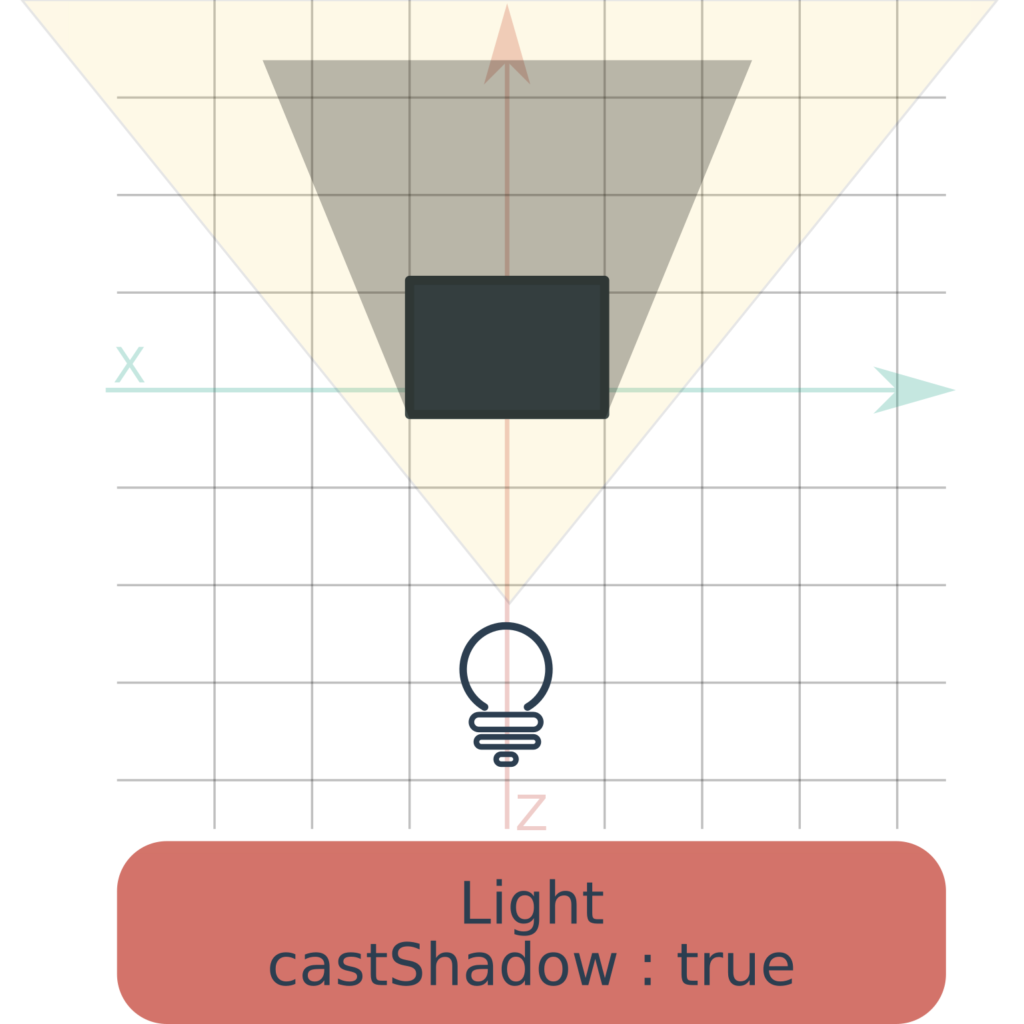
Configure our 3D objects
Receiving a shadow – receiveShadow
When we want to create shadows, it is essential to define a surface able to receive them !
In our example, we will use a Mesh
based on a PlaneGeometry
structure.
//PLANE const planeGeo = new THREE.PlaneGeometry(100, 100); const planeMat = new THREE.MeshPhongMaterial({ color : 'white',side: THREE.DoubleSide}); const meshplane = new THREE.Mesh(planeGeo, planeMat); meshplane.receiveShadow = true; // IMPORTANT FOR SHADOWS !!! meshplane.rotation.x = Math.PI /2; scene.add(meshplane);
The receiveShadow
(Boolean
) property of the Mesh
class defines if the object is able to receive a cast shadow.
To allow our 3D object to receive shadows, we set this value to true
.
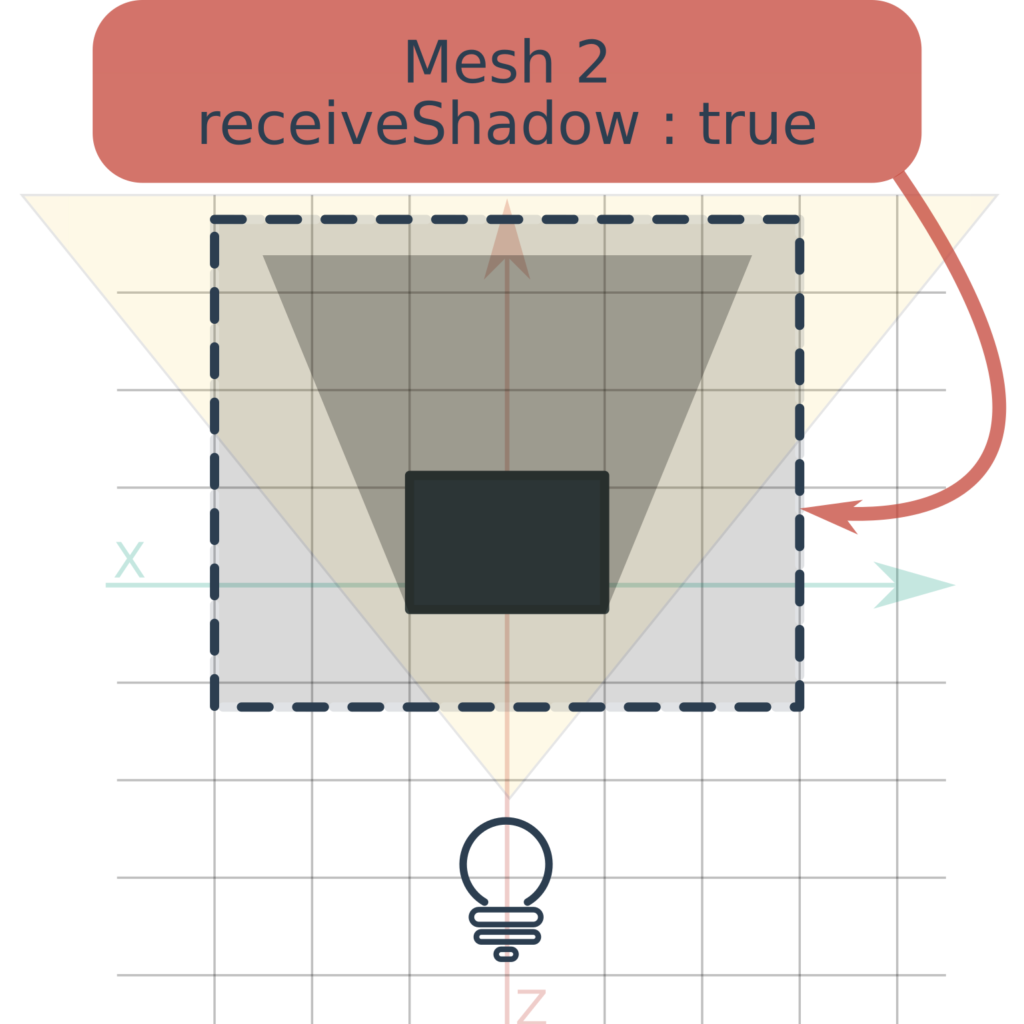
Casting a shadow – castShadow
Finally, let’s create 3D objects that can produce a shadow. The cast shadow will be visible on the surfaces capable of receiving it, as mentioned in the previous paragraph.
To allow a Mesh
to cast a shadow, we use its castShadow
property (Boolean
).
//CUBE const cubeGeo = new THREE.BoxGeometry(2, 2, 2); const cubeMat = new THREE.MeshPhongMaterial({color: '#8AC'}); const meshcube = new THREE.Mesh(cubeGeo, cubeMat); meshcube.castShadow = true; // IMPORTANT FOR SHADOWS !!! meshcube.position.set(5, 1, 5); scene.add(meshcube);
Thus, by setting the value of this property to true
, the concerned object will be able to cast shadows under the effect of an adapted light source.
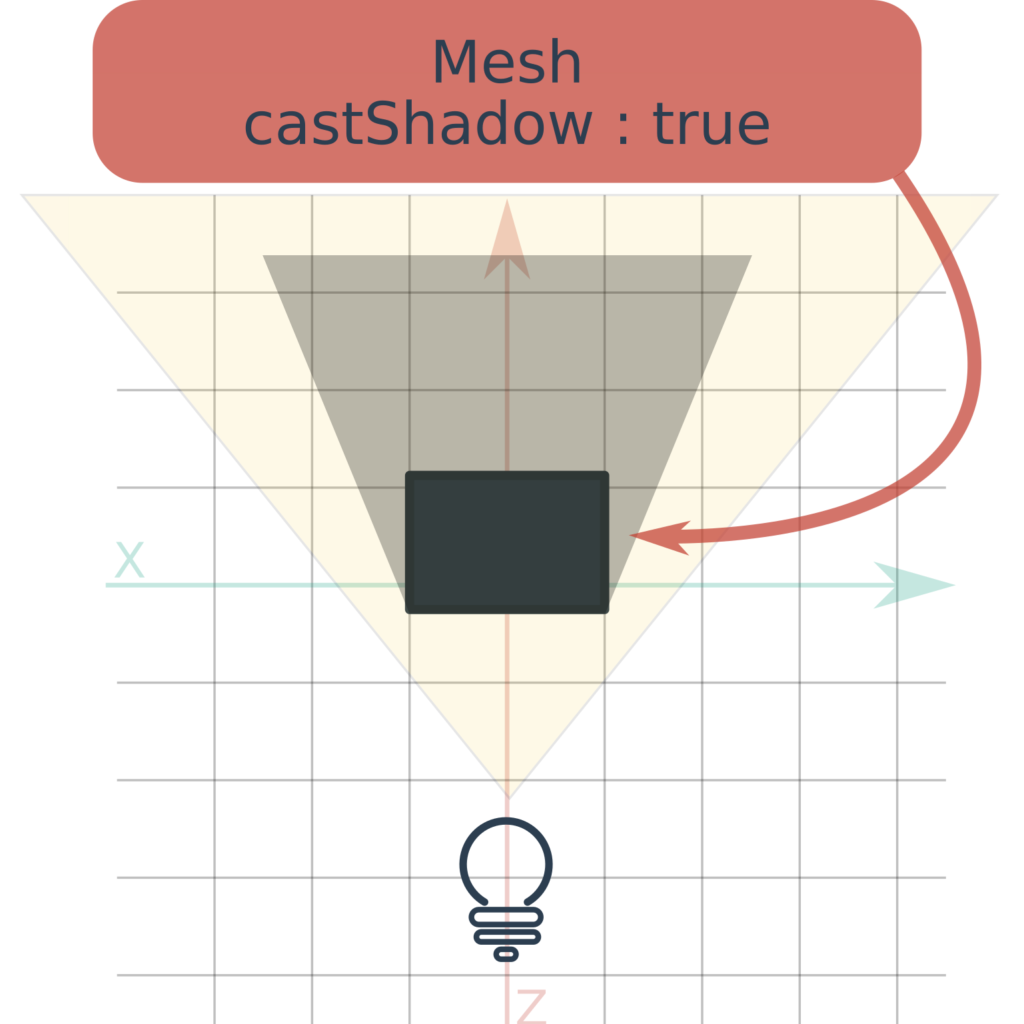
Note that a Mesh
is able to receive and create shadows at the same time : It is possible to set the castShadow
and receiveShadow
properties of the same object to true
!
Final Result
See the Pen Shadows by Thomas (@thomassifferlen) on CodePen.
[…] thomassifferlen 1 commentaire Available in English […]